Avoid browser loading cached api result
Mari Jørgensen
19.05.2021 19:45:20
Recently we had a customer reporting an issue were the content of page was not fully loaded. Initially we had some trouble reproducing this, but with good help from the customer we discovered that the issue only happened when the user navigated back to previous page using the browser back button.
After verifying that page events and scripts were running without errors, we realized that issue was related to the result of an api call.
It turned out that the browser fetched a cached result of an api call (from disk cache) - the result belonging to the previous page.
The solution to this issue was to create a custom ActionFilterAttribute to add to the api method in question.
public class NoCacheAttribute : ActionFilterAttribute
{
public override void OnActionExecuted(
HttpActionExecutedContext actionExecutedContext)
{
var response = actionExecutedContext?.Response;
var headers = response?.Headers;
if (headers == null) return;
headers.CacheControl = new CacheControlHeaderValue
{
NoCache = true,
MustRevalidate = true,
NoStore = true,
};
headers.Add("Pragma", "no-cache");
var contentHeaders = response.Content.Headers;
contentHeaders.Expires = DateTimeOffset.Now;
base.OnActionExecuted(actionExecutedContext);
}
}
And then using that attribute on the api method:
[System.Web.Http.HttpGet]
[NoCache]
public IHttpActionResult MyMethod()
{
}
Kontakt oss her
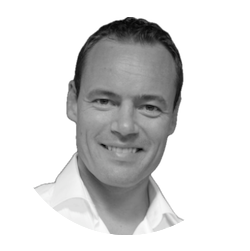