The Meteor Framework is growing up fast, soon it will turn 1.0.
With $11.2 million in funding and a dedicated development group, Meteor is worth paying attention to. In 2012 it was the third most starred open source GitHub repository, and it has had a large community ever since.
What is it?
Writing software is too hard and it takes too long. It's time for a new way to write software ...
The world wide web wasn't built for the awe and wonder we expect from modern day web applications. Like many other frameworks, Meteor addresses this problem. But Meteor is more than just another framework. It is a framework for building apps fast:
What once took weeks, even with the best tools, now takes hours with Meteor.
How to run it?
Mac and Linux: $ curl https://install.meteor.com | /bin/sh
Windows: Install from http://win.meteor.com/
After Meteor has installed, cd
into a directory and run $ meteor create zoo
.
zoo can be anything you'd like, this will be the name of your project.
Run $ cd zoo
then $ meteor
.
Open up http://localhost3000, and you'll see something like this:
This is not a very exiting application so far, what we see is just a simple boilerplate for your project. But it's a good starting point, have a look at the boilerplate code to get an idea of how Meteor works.
How to deploy it?
Run $ meteor deploy zoo.meteor.com
. This is not a good option for high-volume apps running in production. But it is a convenient way of publishing your project to any available .meteor url of your choice, for example http://zoo.meteor.com .
As you'll see in the roadmap, Meteor is developing Galaxy, a commercial service for hosting Meteor apps. Meanwhile, you should consider hosting your app through a NodeJS hosting provider. Run $ meteor bundle zoo.tgz
to export your production-ready application which later can be deployed to your favourite NodeJS host.
The seven principles of Meteor
See http://docs.meteor.com/#sevenprinciples
Data on the Wire.
“Don't send HTML over the network. Send data and let the client decide how to render it.”
Meteor comes with a templating language called Spacebars, which is similar to Handlebars. Other templating languages such as Jade are available as well from Meteor's own package manager https://atmospherejs.com/.
Unlike traditional websites, Meteor downloads the application's HTML templates once. After that, only data is transferred back and forth to the server; in other words, Meteor works like most single page applications.
animals.html
<template name="animals">
<ul>
{{#each species}}
<li>{{name}}</li>
{{/each}}
</ul>
</template>
animals.js
Template.animals.species = function () {
return Animals.find();
};
One Language.
“Write both the client and the server parts of your interface in JavaScript.”
JavaScript on the client and JavaScript on the server. This means that you can write code that runs in both environments, such as in the following example:
var message = 'Hello world';
if (Meteor.isClient) {
console.log(message); // Hello world
}
if (Meteor.isServer) {
console.log(message); // Hello world
}
For real-world projects, code should be split into different directories. The "client" and the "server" directories run code in their respective environemnts. The "lib" directory runs in both, this is a good place to put rules for form validation and Mongo database collections.
Database Everywhere.
“Use the same transparent API to access your database from the client or the server.”
Meteor comes with a client-side JavaScript implementation of Mongo. Forget REST, SQL, Ajax and other middlemen. Communication from the client to the database to the client is seamlessly taken care of by the framework. Write your database queries on the client, set up some permissions server-side, and let the framework handle the rest.
lib/collections.js
// Create a Mongo collection for the
client and the server.
Animals = new Meteor.Collection('animals');
server/server.js
// Publish
Meteor.publish('animals', function(){ return Animals.find(); });
// Security
Animals.allow({
insert: function(userId, doc){
return doc.userId == userId;
},
update: function(userId, doc){
return doc.userId == userId;
},
remove: function(userId, doc){
return doc.userId == userId;
}
});
client/client.js
// Subscribe
Meteor.subscribe('animals');
// Database operations
Animals.insert({name: 'Elephant'});
Animals.update(id, {$set: {weight: 1000}});
Animals.find({}, {sort: {weight: -1}, limit: 5});
Animals.remove(id);
Latency Compensation.
“On the client, use prefetching and model simulation to make it look like you have a zero-latency connection to the database.”
Sending data to the server, saving the data to the database, loading an updated list of records and updating the dom takes time. Meteor skips the roundtrip to the server and updates the dom immediately when the user changes data.
Take the following example:
animals.js
Template.animals.events({ 'submit form': function(){ Animals.update(this._id, {$set: {name: this.name}
, function(error, result){
if(error){
alert('Error!');
}
});
}
});
The user interface will now update before any server response. If the operation fails, the user interface will "roll back" to it's previous state, and an error message will be displayed. And as long as the operation succeed, the user will se the change happen instantaneously.
Full Stack Reactivity.
“Make realtime the default. All layers, from database to template, should make an event-driven interface available.”
Let's imagine that we're looking at a list of animals. What happens when another user changes the name of an animal? The list updates in real time; not the entire page or <ul>, only the <li> that contains the text. Since this process is so unobtrusive, multiple users can easily interact with the same data in real time.
Session is another example of reactivity. Use it for whatever you like, for example HTML attributes, SVG and even in Mongo queries. Or make text dynamic:
animals.html
<template name="animals">
<strong>My favourite animal is {{favouriteAnimal}}.</strong>
<button>Change favourite animal</button>
</template>
animals.js
Template.animals.helpers({
favouriteAnimal: function(){
return Session.get('favouriteAnimal');
}
});
Session.set('favouriteAnimal', 'Elephant');
Template.animals.events({
'click button': function(){
Session.set('favouriteAnimal', 'Giraffe');
}
});
Embrace the Ecosystem.
“Meteor is open source and integrates, rather than replaces, existing open source tools and frameworks.”
Meteor has it's own package manager; https://atmospherejs.com/. Here you'll find a rich selection of Meteor-specific packages, as well as familiar packages such as JavaScript plugins and css frameworks.
Run npm install -g meteorite
to install the package manager.
To add the moment.js package, run $ mrt add moment
then console.log(moment().startOf('day').fromNow();)
Simplicity Equals Productivity
“The best way to make something seem simple is to have it actually be simple. Accomplish this through clean, classically beautiful APIs.”
One example is Meteor's account system.
Run $ meteor add accounts-base
and $ meteor add accounts-password
from your console to set up a complete accounts system.
Run $ meteor add accounts-ui
and add {{> loginButtons}}
to one of your templates:
Run meteor add accounts-github
and meteor add accounts-facebook
:
Clicking those links will walk you through the steps you need to start supporting Github and Facebook authentication.
Run Meteor list
to see other built-in login services.
Add Meteor.users.find({});
to your client-side JavaScript to get all users. Don't worry, Meteor will not send the users` passwords to the client.
Get the currently authenticated user by calling Meteor.user()
, store any data in it's profile
property, controll access by using methods such as
Meteor.users.deny({update: function(){return true;}});
.
And there's more
We've just scratched the surface of Meteor, there's a whole lot more.
Check out Meteor's resources and the documentation which will give you an idea of what's included in the framework.
On Meteor's package manager site you will find a good selection of packages that aren't included in the framework by default.
Also watch this video. It's a bit outdated at the time of writing, but gives a good demonstration of Meteor's principles.
Kontakt oss her
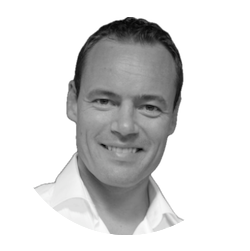